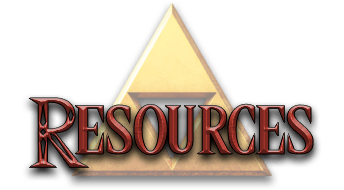
Timers.zh
SubmittedDecember 14, 2016
ZoriaRPG
Timers.zh is intended for users of any level of experience, but it would be best for users who understand how constants work, and how to implement functions in other code.
This library file includes one array, with an index size of '10', and assigns dummy constants to each of these ten elements. You will need to modify this to suit the needs of your game.
Otherwise, the main functions run inside any type of loop (e.g. the main while(true) loop of your global active script), and the functions to set, or re-set the timer, or to check the timer values, can be called from anywhere. This code includes an example global active script.
Set-up of this library is not technically complicated, but can be time-consuming, depending on how many timers you need, and how you want to remember them, and their values, when using functions in instructions.
The included ZIP archive contains a basic list of commands in a text file, with details on usage.
Detailed information on using Timers.zh follows, that assumes you are a novice at ZScript.
A Note About Arrays
This library uses a single array to hold all of its timer values, and the default array has ten (integer) elements. Thus, if you need more than ten timers, you will need to expand the side of the array.
It looks like this:
int Timers[10]={0,0,0,0,0,0,0,0,0,0};
Simply change the value '10' to the value that you need (max 214747), and add additional zeros to the set, separated by commas; save for the last value, that should not have a comma. (See above.)
Next, you will need to define your constants, so that they'll be useful.
Constants Set-Up
The header includes dummy constants for the included array, TV_MYTIMER_1 , to TV_MYTIMER_10 , that you should change to something you will easily remember, that doesn't conflict with other defined constants, or variables. For this reason, it's best to use a prefix--in this case, TI_, for TImer, and later TD for Timer Duration--followed by the name of the timer. TD_DUCK, or TD_EXPLOSION, would be good examples of easy to remember constants.
The number on the right side of these constants matches it to the array, so that you can easily modify the timer values, later.
If you have expanded the size of your array beyond 10 elements, you will want to add more constants for your additional timers here. The first element of an array is '0', and for that reason, TV_MYTIMER_1 = 0 : A reference to TV_MYTIMER_1 is passed as the value '0, when reading from, or writing to the array.
You may assign multiple constants to one index value, either for the purpose of naming conventions (e,g. TV_RED, TV_RUBY), or for other reasons; however you will want to avoid this unless you intend to use either term to reference the same timer.
The next set of constants, is a series of Duration Values, with the prefix 'TD_' (Timer Duration). These are not assigned to the array indices, but rather, they are a count (in frames) for pre-set durations. You may want to establish your own naming here too, but I've provided a list of pre-defined time durations, including fractional-seconds, seconds, and minutes.
You may use these as-is, and utilise multiplications thereof, or combinations thereof, to define durations; else specify a duration directly in an instruction,
Note If you need very long timers, you may need to use tiered timers to expand beyond the ZC MAX_INT limitations.
You will see that the number to the right of these is the length, in frames, for that constant, so if you have any durations that you expect to need commonly, this is the place to add them.
Note: You can manually enter values for timer durations as numbers, when coding instructions.
Using the Libraries
Once you have established your values, you can begin using the functions. The code includes a sample global active script, that runs one timer.
Timer Initialisation
The idea is that for each timer that you have running, you run this pair of functions:
setTimer(TI_MY_TIMER,TD_TIME);
reduceTimer(TI_MY_TIMER);
The first instruction, initiates the timer that you specify as TI_MY_TIMER, with a staring count of TD_TIME.
The second, when running in any loop, that has a Waitframe() instruction at its end, will decrement the same timer by '1' each frame. It is also overloaded to accept a custom value that you may specify:
reduceTimer(TI_MY_TIMER,3);
This would reduce a timer by a value of '3' per frame.
The setTimer() function will not reset the value of the timer when run in a loop, until[/i] that timer reaches '0'; and when that happens, it will automatically reset to the value specified as TD_TIME.
Using Timers
To use these timers, you call upon either the boolean functions checkTimer() and zeroTimer(). The first, checkTimer is less sensitive, and will return 'true' when the timer is at 1, or less; whereas the second, zeroTimer() is strict, and returns 'true' when the timer is exactly zero.
You may call these as any boolean condition:[/i]
if ( checkTimer(TI_MY_TIMER_1 ) {
//Do something
}
Whatever instructions you placed where 'Do something' is noted, would execute when the timer TI_MY_TIMER_1 dropped below a value of '1'.
As an alternative, you may use the function returnTimer() to read the exact value of the timer, and use that as a condition. This can be useful in some circumstances, and you may use this with conditional statements (if, else, else if) similarly to the boolean conditions, or write custom-defined booleans to meet your needs.
Usage Syntax:
checkTimer(TI_TIMER);
zeroTimer(TI_TIMER);
returnTimer(TI_TIMER);
The remaining function allows arbitrary manipulation of timer values.
Example:
changeTimer(TI_MY_TIMER,151);
This would set the timer 'TI_MY_TIMER' to a value of '151', without regard to whatever value it holds at present.
Advanced Use
The above, is the most basic usage of this library, and Timers.zh includes methods of multiple, en-masse timer creation, and manipulation. Please read the enclosed documentation for more information.
This version (v1.5) released on 24th September, 2014, requires no other libraries, or headers.
///////////////////////////////
/// Timers.zh | v1.5 ///
/// 24th September, 2014 ///
////////////////////////////////////////////
/// Created, and maintained by: ZoriaRPG ///
////////////////////////////////////////////
//Array to hold timer values. The index size of each of all of the following arrays MUST be identical.
int Timers[10]={0,0,0,0,0,0,0,0,0,0};
//Expand as needed.
bool TimersRunning[10]={false,false,false,false,false,false,false,false,false,false};
//Expand as needed.
//int TimersStatus[10]={0,0,0,0,0,0,0,0,0,0};
//Reserved for future use. (0 = stopped, 1 = running, 2 = paused).
//Timer Type Constants
//Set up constants for your timers; remember that the array index starts at '0'.
const int TI_MYTIMER_1 = 0;
const int TI_MYTIMER_2 = 1;
const int TI_MYTIMER_3 = 2;
const int TI_MYTIMER_4 = 3;
const int TI_MYTIMER_5 = 4;
const int TI_MYTIMER_6 = 5;
const int TI_MYTIMER_7 = 6;
const int TI_MYTIMER_8 = 7;
const int TI_MYTIMER_9 = 8;
const int TI_MYTIMER_0 = 9;
//Timer Standard Duration Values
const int TD_TENTH_SECOND = 6;
const int TD_QUARTER_SECOND = 15;
const int TD_HALF_SECOND = 30;
const int TD_ONE_SECOND = 60;
const int TD_FIVE_SECONDS = 300;
const int TD_SIX_SECONDS = 360;
const int TD_TEN_SECONDS = 600;
const int TD_FIFTEEN_SECONDS = 900;
const int TD_THIRTY_SECONDS = 1800;
const int TD_1_MINUTE = 3600;
const int TD_2_MINUTES = 7200;
const int TD_3_MINUTES = 10800;
const int TD_4_MINUTES = 14400;
const int TD_5_MINUTES = 18000;
////////////////////////////////////////
//Initialisation and Value Manipulation
void initTimer(int timer, int value){
if ( Timers[timer] <= 0 ) {
Timers[timer] = value;
}
if ( TimersRunning[timer] == false ) {
TimersRunning[timer] = true;
}
}
//Initialises a timer, settign its value, and starting it. If the timer value is not zero, this will fail.
void initTimer(int timer, int value, bool forced){
if ( forced == false ) {
if ( Timers[timer] <= 0 ) {
Timers[timer] = value;
}
}
else if ( forced == true ) {
Timers[timer] = value;
}
if ( TimersRunning[timer] == false ) {
TimersRunning[timer] = true;
}
}
//Initialises a timer, settign its value, and starting it. Can force-set a value.
void setTimer(int timer, int value){
if ( Timers[timer] <= 0 ) {
Timers[timer] = value;
}
}
//Sets the value of a specific timer, if it is zero.
//Used to initialise a timer, and to re-set it onced its count reaches zero.
void setTimer(int timer, int value, bool forced){
if ( forced == false ) {
if ( Timers[timer] 0 ) {
Timers[timer] -= 1;
}
}
}
//Reduce a specific, running timer by '1'. Use before Waitdraw();
void reduceTimer(int timer, int value){
if (TimersRunning[timer] == true ){
if ( Timers[timer] > 0 ) {
Timers[timer] -= value;
}
}
}
//Reduce a specific, running timer by 'value'. Use before Waitdraw();
void clearTimer(int timer){
Timers[timer] = 0;
TimersRunning[timer] = false;
}
//Resets the value of a timer (to zero), and disables it. The opposite of initTimer.
void changeTimer(int timer, int value){
Timers[timer] = value;
}
//Changs the value of a timer to any arbitrary value, with no regard to its present value.
void increaseTimer(int timer, int value){
Timers[timer] += value;
}
//Adds an arbitrary amount to a timer
void shrinkTimer(int timer, int value){
Timers[timer] -= value;
int timerTotal = Timers[timer];
if ( timerTotal == 0 ) {
Timers[timer] = 0;
}
}
//Reduces a timer by an arbitrary amount, even if it is not running. If the reduction would cause the timer to be a negative value, this sets its value to '0'.
void shrinkTimer(int timer, int value, bool ignore){
Timers[timer] -= value;
if ( ignore == false ) {
int timerTotal = Timers[timer];
if ( timerTotal == 0 ) {
Timers[timer] = 0;
}
}
}
//.Reduces a timer by an arbitrary amount, and allows for a ignoring negative values.
//////////////////////////////////////
//Check value and operation of timers.
//Values of Timer
int returnTimer(int timer){
int value = Timers[timer];
return value;
}
//Returns the value of timer as an integer.
//Useful for comparisons, ro events that occur at specific conts of a timer.
bool checkTimer(int timer){
if ( Timers[timer] <= 1 ) {
return true;
}
else {
return false;
}
}
//Boolean returns true when timer reaches '1', or less.
bool checkTimer(int timer, bool precise){
if ( precise == false ) {
if ( Timers[timer] <= 1 ) {
return true;
}
else {
return false;
}
}
else if ( precise == true ) {
if ( Timers[timer] == 0 ) {
return true;
}
else {
return false;
}
}
}
//Boolean returns true when timer reaches 1, or less. May be forced to be sensitive to exactly zero.
bool zeroTimer(int timer){
if ( Timers[timer] == 0 ) {
return true;
}
else {
return false;
}
}
//Boolean returns true when timer reaches exactly '0'.
bool checkTimer(int timer, int value){
if ( Timers[timer] == value ) {
return true;
}
else {
return false;
}
}
//Boolean returns true when timer reaches a specific value.
bool checkTimer(int timer, int value, bool orLess){
if ( orLess == true ) {
if ( Timers[timer] <= value ) {
return true;
}
else {
return false;
}
}
else if ( orLess == false ) {
if ( Timers[timer] == value ) {
return true;
}
else {
return false;
}
}
}
//Boolean returns true when timer reaches a specific value, or less.
///Booleans for Timer Status
bool timerRunning(int timer){
bool value = TimersRunning[timer];
return value;
}
//Returns true is a timer is running, false if 'tisn't.
bool timerOn(int timer){
bool value = TimersRunning[timer];
return value;
}
//Returns true is a timer IS running (ON), false if 'tisn't.
bool timerOff(int timer){
bool tOff;
bool value = TimersRunning[timer];
if ( value == false ) {
tOff = true;
}
else {
tOff = false;
}
return tOff;
}
//Returns true is a timer is NOT running (OFF), false if 'tis.
////////////////////////
//Multiple Timer Actions
///////////////////////////////////
//Reduce - Decrease Multiple Timers
void decreaseAllTimers(int value){
int range = SizeOfArray(Timers);
int presentValue;
for ( int i = 0; 1 0 ) {
Timers[i] -= value;
}
}
}
//Reduces all timers by value specified; will not reduce a timer below zero.
void decreaseAllTimers(int value, bool allowNegative){
int range = SizeOfArray(Timers);
int presentValue;
for ( int i = 0; 1 < range; i++) {
Timers[i] -= value;
presentValue = Timers[i];
if ( allowNegative == false && presentValue < 0 ) {
Timers[i] = 0;
}
}
}
//Reduces all timers, and allows for values below zero.
void decreaseRunningTimers(int amount){
int range = SizeOfArray(Timers);
bool active;
for ( int i = 0; i 0 ) {
Timers[i] -= amount;
}
}
}
}
//Decrease RUNNING timers by arbitrary amount. This will not permnit a timer to drop below zero.
void decreaseRunningTimers(int amount, bool allowNegative){
int range = SizeOfArray(Timers);
bool active;
int presentValue;
for ( int i = 0; i < range; i++ ){
if ( TimersRunning[i] == true ) {
Timers[i] -= amount;
presentValue = Timers[i];
if ( allowNegative == false && presentValue < 0 ) {
Timers[i] = 0;
}
}
}
}
//Decrease RUNNING timers by arbitrary amount. This allows you to permit timers to drop below zero.
void decreaseRangeOfTimers(int min, int max, int value){
for ( int i = min; i 0 ) {
Timers[i] -= value;
}
}
}
//Reduces range of timers, from timer min, to timer max, by amount value.
void decreaseRangeOfTimers(int min, int max, int value, bool allowNegative){
int presentValue;
for ( int i = min; i < max; i++ ){
Timers[i] -= value;
presentValue = Timers[i];
if ( allowNegative == false && presentValue < 0 ) {
Timers[i] = 0;
}
}
}
//Reduces range of timers, from timer min, to timer max, by amount value, with option to permit negative values.
////////////////////////////
//Increase Multiple Timers
void increaseAllTimers(int value){
int range = SizeOfArray(Timers);
for ( int i = 0; 1 0 ) {
Timers[i] += value;
}
}
}
//Increases all timers by arbitrary amount.
void increaseRunningTimers(int amount){
int range = SizeOfArray(Timers);
for ( int i = 0; i < range; i++ ){
if ( TimersRunning[i] == true ) {
Timers[i] += amount;
}
}
}
//Increase all RUNNING timers by an arbitrary amount.
void increaseRangeOfTimers(int min, int max, int value){
for ( int i = min; i 0 ) {
Timers[i] += value;
}
}
}
//Increases range of timers, from timer min, to timer max, by amount value.
////////////////
//Clear Commands
void clearAllTimers(){
int range = SizeOfArray(Timers);
for ( int i = 0; 1 < range; i++) {
Timers[i] = 0;
}
}
//Clears all timers back to a value of zero.
void clearRunningTimers(){
int range = SizeOfArray(Timers);
for ( int i = 0; i < range; i++ ){
if ( TimersRunning[i] == true ) {
Timers[i] = 0;
}
}
}
//Clears all RUNNING timers, back to a value of zero.
void clearSuspendedTimers(){
int range = SizeOfArray(Timers);
for ( int i = 0; i < range; i++ ){
if ( TimersRunning[i] == false ) {
Timers[i] = 0;
}
}
}
//Clears all SUSPENDED timers, back to a value of zero.
void clearPausedTimers(){
int range = SizeOfArray(Timers);
for ( int i = 0; i < range; i++ ){
if ( TimersRunning[i] == false ) {
Timers[i] = 0;
}
}
}
//Clears all PAUSED timers, back to a value of zero.
void clearStoppedTimers(){
int range = SizeOfArray(Timers);
for ( int i = 0; i < range; i++ ){
if ( TimersRunning[i] == false ) {
Timers[i] = 0;
}
}
}
//Clears all STOPPED timers, back to a value of zero.
void clearRangeOfTimers(int min, int max){
for ( int i = min; i 0 ) {
Timers[i] = 0;
}
}
}
//Clears specific range of timers, from timer min,to timer max, back to a value of zero
////////////////
///Set Commands
void setAllTimers(int value){
int range = SizeOfArray(Timers);
for ( int i = 0; 1 < range; i++) {
Timers[i] = value;
}
}
//Sets all timers to an arbitrary amount.
void setRunningTimers(int amount){
int range = SizeOfArray(Timers);
for ( int i = 0; i < range; i++ ){
if ( TimersRunning[i] == true ) {
Timers[i] = amount;
}
}
}
//Sets all RUNNING timers, to an arbitrary value.
void setRangeOfTimers(int min, int max, int value){
for ( int i = min; i 0 ) {
Timers[i] = value;
}
}
}
//Sets specific range of timers, to an arbitrary value.
////////////////////////////////
//Multiple Timer Status Commands
void startAllTimers(){
int range = SizeOfArray(Timers);
for ( int i = 0; 1 < range; i++) {
if ( TimersRunning[i] == false ) {
TimersRunning[i] = true;
}
}
}
//Starts all timers - Call this if you have set multiple timers individually.
void startRangeOfimers(int min, int max){
for ( int i = min; 1 < max; i++) {
if ( TimersRunning[i] == false ) {
TimersRunning[i] = true;
}
}
}
//Starts range of timers, from timer min, to timer max.
void suspendAllTimers(){
int range = SizeOfArray(Timers);
for ( int i = 0; 1 < range; i++) {
if ( TimersRunning[i] == true ) {
TimersRunning[i] = false;
}
}
}
//Suspends all timers.
void suspendRangeOfTimers(int min, int max){
for ( int i = min; 1 < max; i++) {
if ( TimersRunning[i] == true ) {
TimersRunning[i] = false;
}
}
}
//Suspends range of timers, from timer min, to timer max.
void stopAllTimers(){
int range = SizeOfArray(Timers);
for ( int i = 0; 1 < range; i++) {
if ( TimersRunning[i] == true ) {
TimersRunning[i] = false;
}
}
}
//Stops all timers. Reserved for future expansion.
void stopRangeOfTimers(int min, int max){
for ( int i = min; 1 < max; i++) {
if ( TimersRunning[i] == true ) {
TimersRunning[i] = false;
}
}
}
//Stops range of timers, from timer min, to timer max. Reserved for future expansion,
void resumeAllTimers(){
int range = SizeOfArray(Timers);
for ( int i = 0; 1 < range; i++) {
if ( TimersRunning[i] == false ) {
TimersRunning[i] = true;
}
}
}
//Resumes all timers. Reserved for future use with TimersPaused[] array.
void resumeRangeOfTimers(int min, int max){
for ( int i = min; 1 PressR ) {
suspendTimer(TI_MYTIMER_1);
}
else if ( timerOff(TI_MYTIMER_1) && Link->PressR ) {
resumeTimer(TI_MYTIMER_1);
}
//Trace(returnTimer(TI_MYTIMER_1)); //Prints vale of timer TI_MYTIMER_1 to allegro.log
//TraceB(timerRunning(TI_MYTIMER_1)); //Check timer status of TI_MYTIMER_1.
Waitframe();
}
}
}
//Copyright (C) 2014 TMGS
//Licensed Under: GLPL v2 (Read: https://www.gnu.org/licenses/old-licenses/lgpl-2.0.html)
Review this entry
You must be logged in to post a comment.