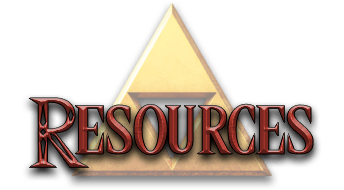
Bolt Spell (or Item) - Thunder & Lightning
SubmittedDecember 14, 2016
ZoriaRPG
Attach this script to a bow, as its Pick-Up script, and when Link gains the bow, he also gains a specified quiver, specific arrows (by type), a number of arrows (or rupees, if not using the 'True Arrows' quest rule). The script will also play a sound, create a hold-up animation, and display a message.
All of the following are configurable with arguments:
The quiver to award (if any).
The arrow item to award (if any).
The number of arrows to award (if any).
The sound to play (if any).
The message to display (if any).
The Hold-Up-Item animation to use (if any).
You may enable any, or all of these features at your discretion. The script automatically increases the arrows MCounter (maximum) based on the value assigned as the 'increase Counter Max' entry in the item editor properties for the quiver you choose to give (if any). if this value is lower than the present max arrows, the higher of the two values is always used.
The script uses intelligent boolean control, so that it only awards what you desire to award. You may award a bow, and a quiver, but no arrow item, or if you gave the player a quiver in init data, you may award only the bow, and an arrow type, or any combination of the options above.
The example quest demonstrates awarding a small quiver, and wooden arrows with the shortbow; and on the next screen, awarding the longbow, with a medium quiver, and silver arrows.
Please see the example quest, and documentation in the package.
The longbow, in the example quest (on screen 01), is packaged with the silver arrows. These silver arrows are cheaply set-up to use rupees in addition to arrows, just as a demonstration of using a negative value for arg D0 for quests with 'True Arrows' disabled.
If you are not using any other scripts, uncomment the first line: ' import "std.zh" '.
Add the script to your ZC buffer, or use an import directive to add it.
Compile, and assign the item script BowPickup to an item script slot.
If desired, create a string to display for this pick-up in Quest->Strings.
If desired, add a custom sound for this pick-up in Quest->Audio->SFX Data
Go to the item editor, and select a bow, then open it in the editor.
Attach the script 'BowPickup' to the item's Pick-Up slot on the 'Scripts' tab.
While still in the item editor, note the item numbers for the arrow type (e.g. silver), the quiver item, and the item number of the bow itself. A series of default values are listed in the script comments for easy access.
Script Arguments
While on the 'Scripts' tab of the bow item attributes pages of the Item Editor...
Set up the arguments (D0, D1, D2, D3, D4, D5, and D6) as follows:
D0: A number of starting arrows to give the player with the bow.
Setting this to '0' will award no arrows.
Setting it to a positive value (1 or more), will award arrows equal to the number.
Setting it to a NEGATIVE number ( e.g. -20) will award a value in rupees equal to that number, if it was positive (e.g. -20 gives 20 rupees). This is for legacy style games that use rupees as ammo.
D1: The Quiver to give the player, if desired.
If you wish to award a quiver, enter the item number for it here.
If you are not using the 'True Arrows' quest rule, you do not need to award a quiver.
Note: You may leave this blank ( 0 ), when not using 'True Arrows'. Otherwise, leave it blank only if you either do not wish to award a quiver, or if the player already has one. Without a quiver, no arrows will be awarded, and the player will be unable to pick up arrow ammo drops, or use the arrow item.
D2: The type of arrow to award:
If the player already has an arrow type, and you do not wish to upgrade it, you may leave this blank ( 0 ).
Otherwise, add the item number of the arrow type that you wish to award here.
D3: The sound effect to play when the player is given the item bundle.
The standard 'Special Item' sound in ZC is '20'.
If you leave this blank ( 0 ), it will not play a sound.
D4: The message string (from Quest->Strings) ID number to display when awarding the player a bundle.
If you do not wish to display a string, set this value to '0'.
Otherwise, assign the value of the String ID that you wish to display.
D5: Set to a value of '1' or higher, to cause Link to hold the item over his head.
Values:
0: Do not hold above head.
1: Holds with one hand.
2: holds with two hands.
3: Holds with one hand, as a Dive (water) secret
4: Holds with two hands, as a Dive (water) secret.
D6: If you are causing the player to hold the item overhead, set this to the item ID number of the bow that you are awarding. You can actually specify any item number here, and Link will hold that overhead. If you, as an example, want to make a special item that has the bow, and arrow together (not matching what you place on your subscreen), you can call the ID of that here.
Please see the values in the example quest if you need a reference.
The distributed package includes a text file with a copy of these set-up instructions.
Requires: std.zh
import "std.zh"
////////////////////////////////////////////
/// Bow, Arrow, and Quiver Bundle Pickup ///
/// v0.2.1 - 9th July, 2015 ///
/// By: ZoriaRPG ///
////////////////////////////////////////////
// Script Arguments
// D0: Number of starting arrows to give with this bow.
// D1: Quiver to Give: Small Quiver ( 74 ), medium Quiver ( 75 ), Large Quiver ( 76 ), Magic Quiver ( 105 ) , or custom.
// D2: Type of arrow to give with bow: Wooden ( 13 ), Silver ( 14 ), Gold ( 57 ), or custom.
// D3: Sound Effect to play: Special Item ( 20 ), or custom.
// D4: Message String ID to display (if any). None ( 0 ), or custom.
// D5: Hold-Up Animation to use: None ( 0 ) , 1-Hand ( 1 ), 2-Hand ( 2 ) , 1-Hand-Water ( 3 ), 2-Hand-Water ( 4 ).
// D6: Bow to give: Bow ( 15 ) , Longbow ( 68 ), or custom. This should match the item ID to which you attach this script!
item script BowPickup{
void run(int giveArrows, int quiver, int arrowType, int playSFX, int showMessage, int holdUp, int bowID){
int arrowsMax;
if ( quiver && !Link->Item[quiver] ) {
Link->Item[quiver] = true;
itemdata quiverIT = Game->LoadItemData(quiver);
arrowsMax = quiverIT->Max;
}
if ( Game->MCounter[CR_ARROWS] MCounter[CR_ARROWS] = arrowsMax;
if ( arrowType && !Link->Item[arrowType] ) Link->Item[arrowType] = true;
if ( giveArrows ) Game->Counter[CR_ARROWS] += giveArrows;
if ( giveArrows Counter[CR_RUPEES] -= giveArrows;
if ( playSFX ) Game->PlaySound(playSFX);
if ( holdUp && bowID ) _Bow_HoldUpItem(bowID,holdUp);
if ( showMessage ) Screen->Message(showMessage);
}
}
//Global Constants and Functions
//Item Hold-Up Constants
const int ITM_HOLD1LAND = 1;
const int ITM_HOLD2LAND = 2;
const int ITM_HOLD1WATER = 3;
const int ITM_HOLD2WATER = 4;
//Global Function to force Link to hold up any item specified as itm, using animation specified by holdType.
void _Bow_HoldUpItem(int itm, int holdType){
if ( holdType == ITM_HOLD1LAND ) {
Link->Action = LA_HOLD1LAND;
Link->HeldItem = itm;
}
if ( holdType == ITM_HOLD2LAND ) {
Link->Action = LA_HOLD2LAND;
Link->HeldItem = itm;
}
if ( holdType == ITM_HOLD1WATER ) {
Link->Action = LA_HOLD1WATER;
Link->HeldItem = itm;
}
if ( holdType == ITM_HOLD2WATER ) {
Link->Action = LA_HOLD2WATER;
Link->HeldItem = itm;
}
}
Review this entry
You must be logged in to post a comment.